Multiple Fonts in Next.js 13
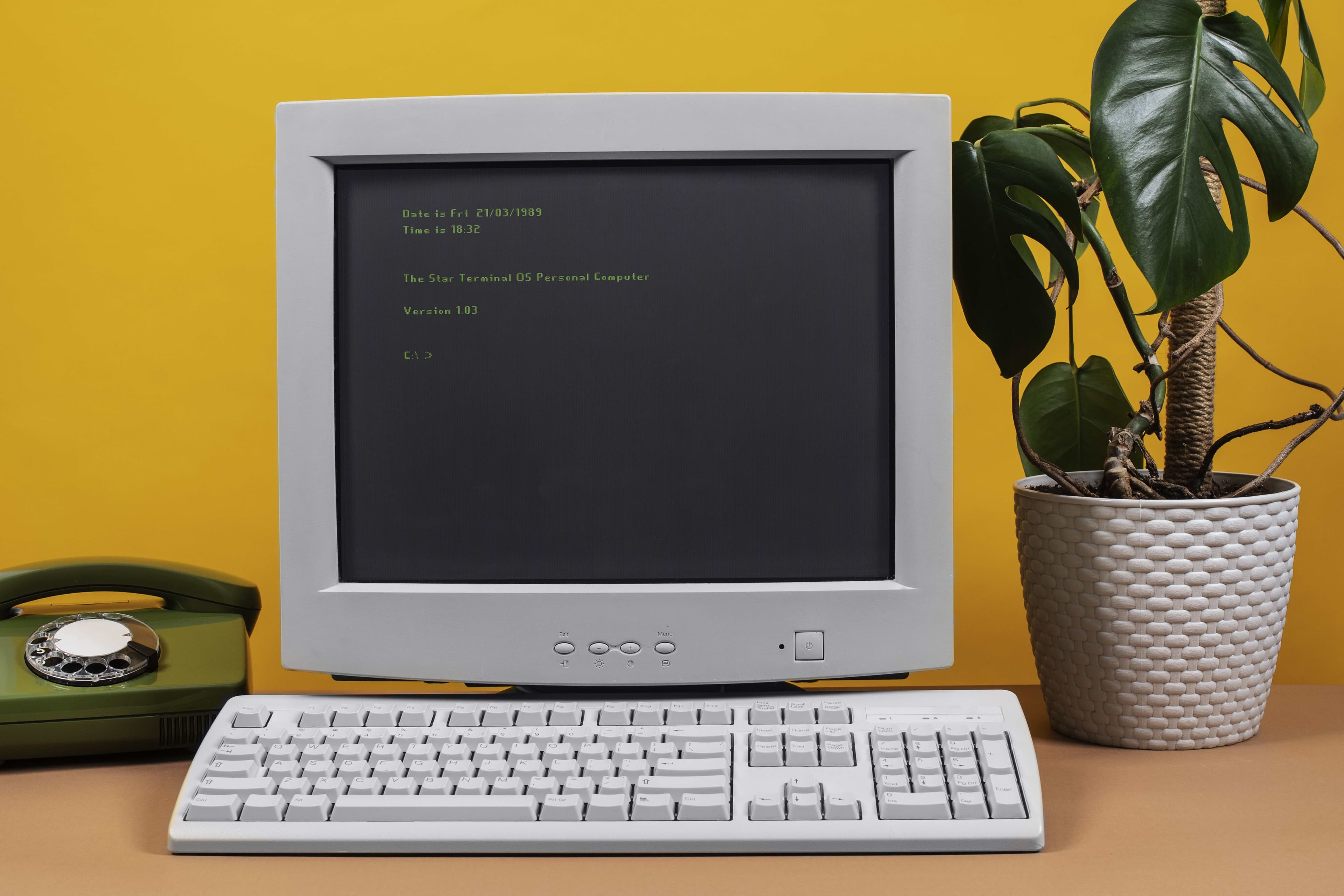
June 27, 2023
When working on a project with Next.js, one of the exciting features to explore is the Font Module, which optimizes fonts for enhanced privacy and performance. The official Next.js documentation provides a comprehensive guide on utilizing this module, including instructions for using multiple fonts .
However, there’s an inconvenience that arises when working with the Font Module. By default, Next.js recommends using variables within the className prop, which can become messy and impair code readability, when dealing with more than one class.
The example in the documentation looks pretty clean:
<h1 className={roboto_mono.className}>My page</h1>
But things get really complex once you want to add more classes, transforming it into an interpolated string for example when using Tailwind CSS for styling:
<h1 className={`${roboto_mono.className} text-4xl font-bold text-zinc-800`}>
My page
</h1>
Yes, the code is still valid, but I find it extremely inconvenient to write {`${}`} for every text line. So today’s goal is to apply the font with a string in className.
Here is how to achieve it step-by-step:
- In 'layout.tsx' import fonts from ‘next/font/google’ (or ‘next/font/local’). Create constants for the font names. Make sure to include CSS variables for each font.
import "./globals.css";
import {Advent_Pro, Capriola} from "next/font/google";
const capriola = Capriola({
subsets: ["latin"],
weight: "400",
variable: "--font-capriola",
});
const adventPro = Advent_Pro({
subsets: ["latin"],
weight: "400",
variable: "--font-advent-pro",
});
- Add the font variables as classNames to the parent tag in your layout component.
export default function RootLayout(
{children}: { children: React.ReactNode; }) {
return (
<html lang="en" className={`${capriola.variable} ${adventPro.variable}`}>
<body>{children}</body>
</html>
);
}
- In your global stylesheet (e.g., globals.css), create classes for the fonts and apply any additional font styles if needed. Use variable names defined in the previous step.
.text1 {
font-family: var(--font-capriola);
}
.text2 {
font-family: var(--font-advent-pro);
font-weight: 200;
color: #5c5cff;
}
- Now, in your components and pages, you can simply add classes to apply the font family and styling.
export default function Home() {
return (
<>
<h1>Default</h1>
<h1 className="text1">Capriola</h1>
<h1 className="text2">Agdasima</h1>
</>
);
}
That’s how it is going to look in the browser:
By following these simple steps, you can conveniently apply the fonts using classes in Next.js without the need for
string interpolation in every line, making your code more readable.
For the full code together check out GitHub.